Overview
This project explores using the GR-PEACH board with ROHM sensor evaluation kits. The kits enable the use of eight sensors - an accelerometer, pressure sensor, magnetometer, ambient light proximity sensor, color sensor, Hall IC, temperature sensor, and an ultraviolet sensor. The project will introduce how to use these kits with GR-PEACH and the web compiler.
Preparation
You will need a GR-PEACH board, USB cable (Micro B type) and ROHM sensor evaluation kits.
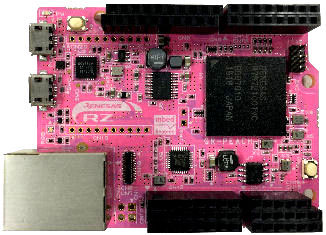
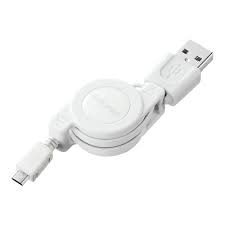
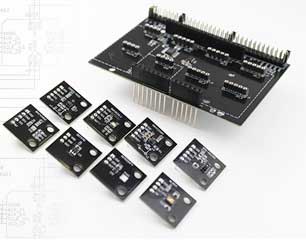
Importing the Libraries
Downloading
Download the rohm-lib zip file below which will include libraries for the accelerometer, pressure sensor, ambient light proximity sensor, color sensor, temperature sensor, and ultraviolet sensor. The libraries are also on the Rohm website.
Download the Rohm Sensor Libraries File- Rohm Sensor Libraries (ZIP)
Uploading to the Web Compiler
First, create a project for GR-PEACH on the web compiler. Then, upload the zip into the root of your project. For more information on how to create a project using the web compiler, refer to the Sketch on Web Compiler project.
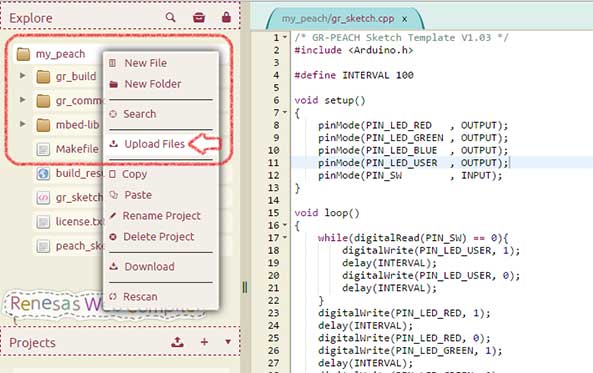
The folder "rohm-lib" is generated as well as the library files.
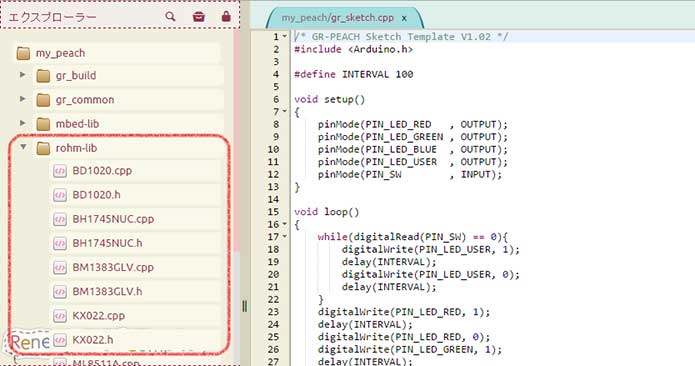
Connecting the Hardware
Connect five sensor modules to evaluation kits and set the J15 jumper to 3V.
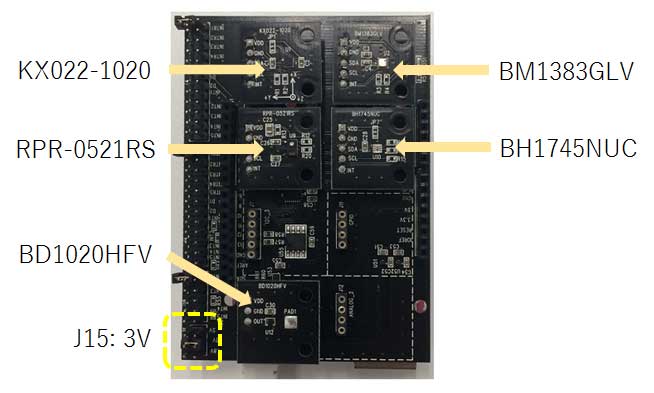
Connect the kits to GR-PEACH. Then, connect GR-PEACH to a PC with the USB cable. This completes the connection of all of the hardware.
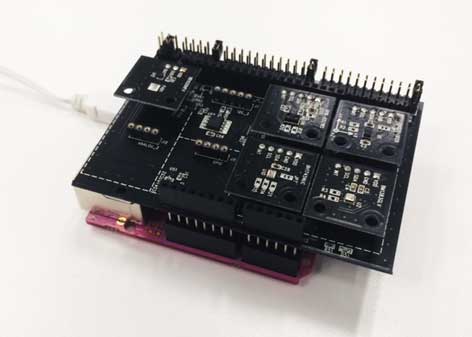
Sample Program
The sample below outputs the value of the five sensors. Please monitor using software such as Tera Term or CoolTerm. Copy the sample to gr_sketch.cpp on the web compiler and then build the sketch.
#include <Arduino.h>
#include <Wire.h>
#include <KX022.h>
#include <BM1383GLV.h>
#include <RPR-0521RS.h>
#include <BH1745NUC.h>
#include <BD1020.h>
#include <ML8511A.h>
KX022 kx022(KX022_DEVICE_ADDRESS_1E); //Accelerometer
BM1383GLV bm1383; //Pressure Sensor
RPR0521RS rpr0521rs; //PS/ALS Sensor
BH1745NUC bh1745nuc(BH1745NUC_DEVICE_ADDRESS_39); //Color Sensor
BD1020 bd1020; //Temperature Sensor
ML8511A ml8511a; //UV Sensor
int tempout_pin = 14; // A0
int uvout_pin = 16; // A2
void setup() {
Serial.begin(9600);
Wire.begin();
kx022.init();
bm1383.init();
rpr0521rs.init();
bh1745nuc.init();
bd1020.init(tempout_pin);
ml8511a.init(uvout_pin);
}
void loop() {
byte rc;
float acc[3], press, als_val, temp, uv;
unsigned short ps_val, rgbc[4];
byte near_far;
//Accelerometer
rc = kx022.get_val(acc);
if (rc == 0) {
Serial.print(" x= ");
Serial.print(acc[0]);
Serial.print(" y= ");
Serial.print(acc[1]);
Serial.print(" z= ");
Serial.print(acc[2]);
}
//Pressure Sensor
rc = bm1383.get_val(&press);
if (rc == 0) {
Serial.print(" ");
Serial.print(press);
Serial.print("[hPa]");
}
//PS/ALS Sensor
rc = rpr0521rs.get_psalsval(&ps_val, &als_val);
if (rc == 0) {
Serial.print(" ");
Serial.print(ps_val);
Serial.print("[cnt]");
near_far = rpr0521rs.check_near_far(ps_val);
Serial.print("->");
if (near_far == RPR0521RS_NEAR_VAL) {
Serial.print(F(" Near"));
} else {
Serial.print(F(" Far"));
}
if (als_val != RPR0521RS_ERROR) {
Serial.print(" ");
Serial.print(als_val);
Serial.print(F("[lx]"));
}
}
//Color Sensor
rc = bh1745nuc.get_val(rgbc);
if (rc == 0) {
Serial.print(" R:");
Serial.print(rgbc[0]);
Serial.print(" G:");
Serial.print(rgbc[1]);
Serial.print(" G:");
Serial.print(rgbc[2]);
Serial.print(" C:");
Serial.print(rgbc[3]);
}
//Temperature Sensor
bd1020.get_val(&temp);
Serial.print(" ");
Serial.print(temp);
Serial.print("[C]");
Serial.println();
delay(100);
}
When the sample operates successfully, the value of sensors are displayed in the serial monitor.
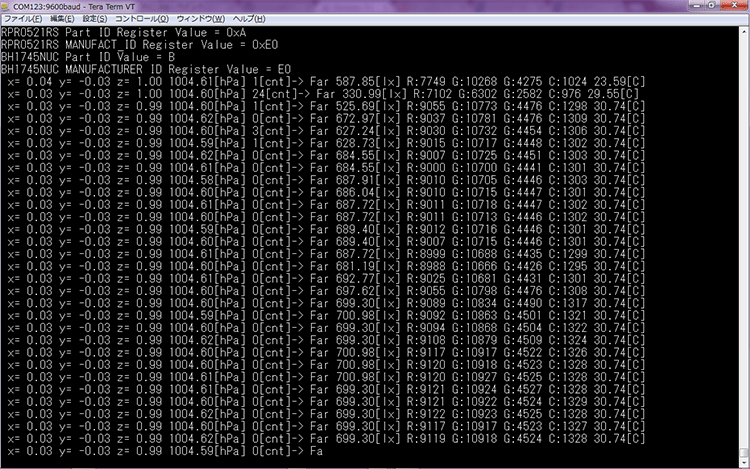